How to Make an AJAX Request Without Using Frameworks or Libraries
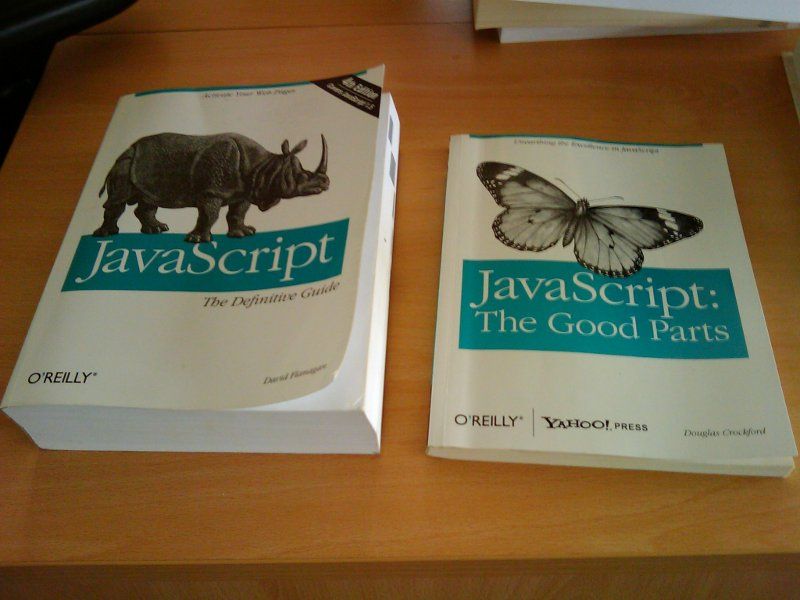
There are a lot of JavaScript frameworks out there these days. It makes it harder to choose a front-end development workflow when starting a new project and write code that’s portable between different projects. Also, using a complex framework to solve a simple problem adds unneeded complexity and risk to a project, which makes getting that project out the door slower and more troublesome.
In the olden days, which in internet years was around 2008, browsers were horribly inconsistent. What worked in Internet Explorer wouldn’t work in Firefox. Chrome was still just a glimmer in Google’s eye. Using frameworks to perform simple tasks such as querying the DOM, performing animations, and making AJAX requests was required if you didn’t want to spend time rolling your own solution.
The downside to this was that relying on frameworks and libraries for every front end web development project has been cargo-culted through the years, despite that fact there have been a ton of advancements with browsers. (side note, if you’re ever unsure if browsers support a particular feature, check caniuse.com.)
Here’s a simple function I used in a recent project to handle AJAX requests. I took this approach because I was working on a background page of a Google Chrome extension, and felt that shoehorning a framework in to handle a few small tasks would be overkill. Here’s what I came up with:
function ajax(method, url, success, failure) { var xhr = new XMLHttpRequest(); xhr.open(method, url, true); xhr.onreadystatechange = function() { if(xhr.readyState == XMLHttpRequest.DONE) { if(xhr.status >= 200 && xhr.status <= 299) { success(xhr.response); } else { failure(xhr.response); } } } xhr.send(); return xhr; }
(Edit: Shout out to Matt Parsons for helping me improve this function based on a conversation from the Classic City Devs Slack Channel.)
The function is code takes four arguments: HTTP method, URL of the request, a success callback, and a failure callback.
Now, if I wanted to query and get some adorable animals from Reddit, I can do so in pure VanillaJS. Here’s an example:
function doStuff(response) { ... } function handleError(response) { ...} var url = 'https://www.reddit.com/r/aww.json'; ajax('GET', url, doStuff, handleError);
Think before you npm install
. Don’t include thousands of lines of a framework when you can solve the solution in a dozen lines of native JavaScript.